What is Point-to-Point?
Point-to-point is the most commonly used communication pattern in MPI. It involves the transfer of a message from one specific process to another specific process in the communicator. The point-to-point pattern requires action from both the sending and receiving processes. The sending process must execute some form of MPI_SEND, and the receiving process must execute some form of MPI_RECV.
Both processes must know:
- with whom they are communicating, i.e., the source or destination of the message,
- the message-identifying tag associated with the message, and
- the type and the amount or size of data that are being passed.
This simple process, which we will build on later, can be illustrated as follows:
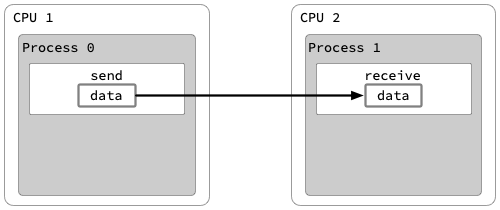
MPI provides both blocking and nonblocking point-to-point communication:
- Blocking communication means that the process waits to ensure the message data have achieved a particular state before processing can continue.
- Nonblocking communication means that the processor merely requests to start an operation and continues processing.
A blocking call is "safer" in that it ensures the message data are ready to be used — read by the receiver or modified by the sender — once the call returns. However, the data of the message may not need such protection until later in the program; if that is the case, then a nonblocking call can be used instead. The pseudo-code below demonstrates the general difference:
The issue we've avoided in the above is: what exactly will make the blocking call stop blocking? It might be reasonable to assume that the SEND blocks until the message is successfully received by the RECV-ing process. However, MPI doesn't work quite that way. Generally speaking, blocking calls return when the sending buffer (i.e., the array or variable name where the message is stored) can be safely modified, which means only that the data have been moved to some other location (note we don't specify where that location is). The next section will cover the four available communication modes, how they operate, and how they impact when blocking calls can return.