Broadcast
In many instances, there is one process that needs to send (broadcast) some data (either a scalar or vector) to all the processes in a group. MPI provides the broadcast primitive MPI_Bcast to accomplish this task. The syntax of the MPI_Bcast call is:
Broadcast Syntax
Parameters for Broadcast routine:
buffer
- is the starting address of a buffer
count
- is an integer indicating the number of data elements in the buffer
datatype
- is an MPI-defined constant indicating the data type of the elements in the buffer
root
- is an integer indicating the rank of the broadcast root process
comm
- is the communicator
The MPI_Bcast must be called by each process in the group, specifying the same comm and root. The message is sent from the root process to all processes in the group, including the root process. If we think in terms of a matrix whose rows and columns correspond to the data and processes, respectively, then the states of this matrix before and after the call can be illustrated as follows:
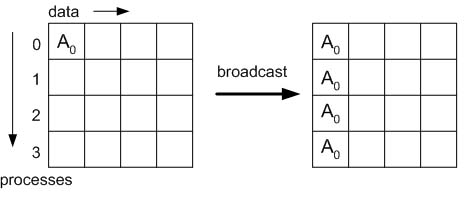
The "Hello, world" example used in an earlier roadmap could have used broadcast instead of multiple sends and receives: