Programmable Source
ParaView's Programmable Source allows users to write a Python script that generates visualization pipleline data. A common use of a Programmable Source is to read data from a custom format into the visualization pipeline. They can also be used to generate data algorithmically.
As discussed in the Python Shell section,
ParaView includes a Python wrapper for its lowest level functionality,
the Visualization Toolkit (VTK).
Programmable Source scripts use the classes and methods exposed in this Python VTK wrapper
to add data to the visualization pipeline.
While it is possible to import and use the functionality of
the paraview.simple
module in a Programmable Source,
the finer-grained functionality of the paraview.vtk
module is generally required.
Descriptions of its classes can be found in
the C++ VTK documentation.
Programming against this API is easier than writing a C++ ParaView plugin,
but more difficult than writing a Python Calculator expression.
Under the covers in ParaView, a Programmable Source is actually an instance of the VTK's vtkProgrammableFilter class. When you create a Programmable Source in ParaView, the script text you enter is injected into a skeleton Python method that is then assigned to be the "execute" method of the host vtkProgrammableFilter object. This method is then invoked as needed by the VTK.
There is no need to import the paraview.vtk
module in your Python script text,
as it is automatically included in the skeleton method by the Programmable Source.
VTK classes can be instantiated using vtk
methods.
Your script can access the methods of the host vtkProgrammableFilter instance through the self
variable.
The ParaView Reference Manual contains a section on writing these scripts.
If you download the VTK software,
it contains numerous example scripts that are very instructive.
Creating a Programmable Source
Let's explore the Programmable Source with a script that creates a four sided polyhedron. We begin by creating and initializing a Programmable Source:
- Edit→Reset Session
- Sources→Alphabetical→Programmable Source
- In the Properties panel, Output Data Set Type must be set to "vtkPolyData" for this example.
- Copy the following script and paste it into the Script section, then click "Apply".
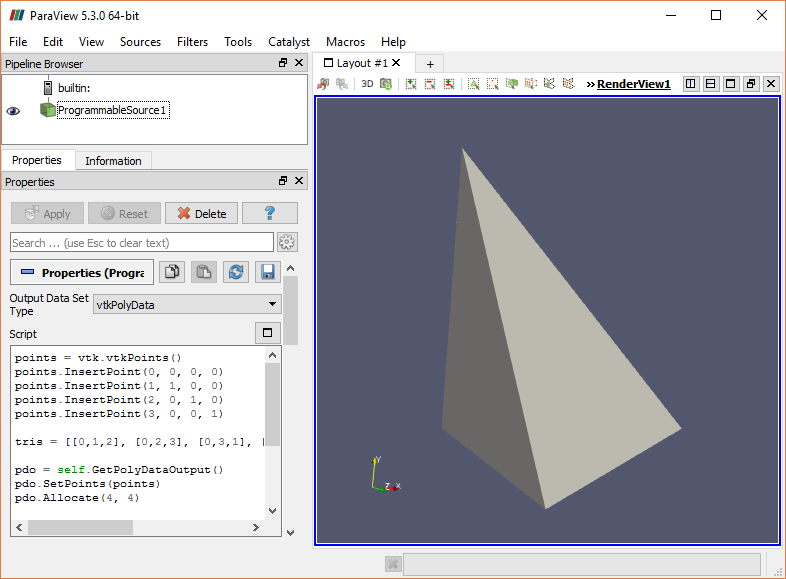
The first block of code in this script asks the VTK library to allocate an empty point collection. Four points (the corners of our polyhedron) are then added to this collection. Later, we will be adding triangles to the visualization pipeline, and those triangles are defined as sets of indices into this point collection.
Next, we use the self
object
to access the functionality of the vtkProgrammableFilter class.
After accessing the output object for polygonal data,
we must assign both the point and cell elements that define the polygonal data.
We assign our previously allocated point collection
and then allocate four cells in the object.
For polygonal data sets each cell defines a polygon,
while for grid data sets each cell is a cuboid bounded by the eight points at its corners.
The remaining code in the script creates the triangles in this polyhedron. First, we ask the VTK library to instantiate a single polygon object, which we will repeatedly fill with data and add as a cell to the output polygonal object. Since all of the polygons we are creating are triangles, we can assign its size, or "number of IDs", just once. Then, for each of the four triangles, we copy three vertex indices into the triangle object and add the triangle as the next "cell" in the object. When we clicked "Apply" the script was executed and the display was refreshed, causing the polyhedron to appear.
If there are problems with the script of the Programmable Source, the "Output Messages" window will automatically be shown when you click "Apply" and error messages will be displayed there. This window can be shown manually using View→Output Messages.
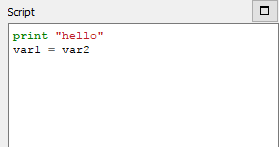
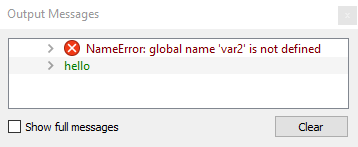
Printed output from the script will also be shown in this window. This is a useful debugging tool if you are having trouble with a script. Note that if a script contains a serious enough error, it is possible for ParaView to crash when it executes the script. After making large changes, be sure to make a backup copy your script text before clicking "Apply"!